OPS445 Assignment 2
Assignment 2 - Load Balancer Monitor
In this assignment you will create the beginning of what could theoretically one day become a monitoring system for a load balancing setup.
The general idea is that you'll have a LoadBalancer object representing a single subnet, which will contain some number of Node objects. When it's all working, you'll be able to:
- Add/remove slaves to/from your load balancer.
- Call a single LoadBalancer function to check the status of the entire setup, and print a summary for you.
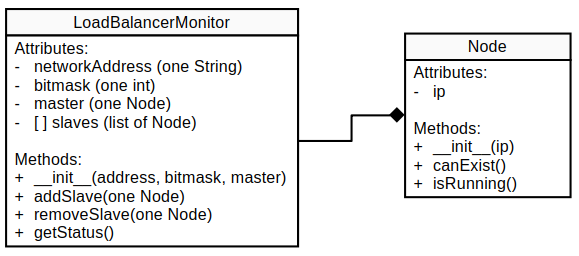
The requirements are minimal, and are missing much functionality and error checking to be usable as a real monitoring system, but hopefully doing this assignment you'll get an idea of how Python could be useful in a scenario like this.
Header
Your code must be a single source file named check_apache_log_yourmysenecaid.py, and at the top of that file it will contain the following comment:
# OPS435 Assignment 2
# load_balancer_monitor_yourmysenecaid.py
# Author: Your Name
Node class
An object of this class can represent a computer, a virtual machine, or whatever other device your load balancer might use.
Following is a list of attributes and methods for this class:
ip attribute
Stores the IP address for the node, as a String.
No subnet mask is stored, to make your work for this assignment a little easier.
__init__(ip) method
Builds a node. Receives a string representation of the node's IP address.
canExist() method.
Receives no arguments.
Checks whether the IP address stored in this node is a valid IP address:
- Has exactly four octets, delimited with a dot.
- The first octet cannot be zero.
- The last octet cannot be zero.
- Every octet must be a number greater or equal to zero, and less than 255.
If all the checks pass: canExist() should return True.
If any of the checks fail: canExist() should return False.
isRunning() method
Receives no arguments.
Simply ping the ip address stored in the object, waiting a maximum of two seconds for a reply.
The easiest way to do this is the subprocess.getstatusoutput() function, similar to the subprocess.Popen() you've used in Lab 3. Call the following shell command and check the return code:
ping -W 2 -c 1 IPADDRESSOFNODE
If the ping commands succeeds (i.e. returns zero): isRunning() should return True.
If the ping command fails (i.e. returns any value other than zero): isRunning() should return False.
LoadBalancerMonitor class
An object of this class is meant to monitor your entire load balancer setup: one master and a number of slaves.
Following is a list of attributes and methods for this class:
networkAddress attribute
Stores the network address for the subnet, as a String.
bitmask attribute
Stores the bitmask for the network, as an int.
master attribute
Stores the node on your subnet which is used as the master, as an object of the Node class.
To make the assignment simpler: no need to check whether the node's ip address is in the subnet.
slaves attribute
A python list of Node objects, each of which has the role of a slave.
__init__(networkAddress, bitmask, master) method
Builds a LoadBalancerMonitor object.
Receives and stores in the respective attributes:
- networkAddress as a String
- bitmask as an int
- master as a Node
To make the assignment simpler: no need to do error checking on these constructor arguments.
addSlave(node) method
Receives an object of type Node representing a slave.
If the node canExist(): adds it to the slaves list. Otherwise just prints an error message.
To make the assignment simpler: no need to do error checking on the node argument.
removeSlave(slave) method
Receives an object of type Node.
Checks whether this slave exists in the slaves list, and if it does: removes it from the list.
getStatus() method
Receives no arguments.
Prints a string in the following form:
Checking....... Load Balancer Status ==================== Master: ONLINE/OFFLINE Slaves: X ONLINE, Y OFFLINE
With one dot following "Checking" for each node that's being checked. You might want to look into the flush argument for the print function if the output doesn't function exactly as you expect.
The master status should be online if the master's canExist() and isRunning() method sreturn True.
The slaves' status should be determined by counting how many of the slaves appear to be running (by calling each of the slaves' isRunning() method.)
Does not return anything.
Testing
You should come up with your own code to test whether your classes are implemented correctly. But you can also use any parts of the following if you like:
ip1 = Node("0.168.0.1")
print(ip1.canExist()) # Should print False
ip1 = Node("192.168.0.0")
print(ip1.canExist()) # Should print False
ip1 = Node("192.168.1")
print(ip1.canExist()) # Should print False
ip1 = Node("192.268.0.1")
print(ip1.canExist()) # Should print False
ip1 = Node("10.4.45.100")
print(ip1.canExist()) # Should print True
print("ip1.ip is running: " + str(ip1.isRunning())) # Should print ip1.ip is running: False
master = Node("1.1.1.1") # For easy testing
print(master.canExist()) # Should print True
print("master.ip is running: " + str(master.isRunning())) # Should print master.ip is running: True
monitor = LoadBalancerMonitor("1.1.1.0", 24, master)
slave = Node("127.0.0.0")
monitor.addSlave(slave) # Should print an error message
slave = Node("127.0.0.1") # For easy testing
monitor.addSlave(slave)
slave = Node("127.0.0.2") # For easy testing
monitor.addSlave(slave)
slave = Node("127.0.0.3") # For easy testing
monitor.addSlave(slave)
slave = Node("10.4.45.100") # For easy testing
monitor.addSlave(slave)
slave = Node("127.0.0.2") # For easy testing
monitor.addSlave(slave)
monitor.removeSlave(slave)
# The following should print:
# Checking.....
#
# Load Balancer Status
# ====================
# Master: ONLINE
# Slaves: 3 ONLINE, 1 OFFLINE
monitor.getStatus()
Submission
After testing your program - submit the load_balancer_monitor_yourmysenecaid.py file via Blackboard. Don't submit another document, nor a screenshot, nor an archive.