OPS145 Lab 4
Binary review
Go back and review the binary stuff we looked at in the course introduction.
This lab is about POSIX permissions, and you need to be completely comfortable with binary-to-decimal and decimal-to-binary conversion from 000 to 111 (decimal 0 to 7).
Here's a summary of the absolute minimum you need to remember from that:
Binary | Decimal |
---|---|
000 | 0+0+0=0 |
001 | 0+0+1=1 |
010 | 0+2+0=2 |
011 | 0+2+1=3 |
100 | 4+0+0=4 |
101 | 4+0+1=5 |
110 | 4+2+0=6 |
111 | 4+2+1=7 |
Read, Write, Execute (rwx) permissions
On a Linux filesystem every file and directory has 9 bits of information allocated for reording basic permissions. Those 9 bits are split into three groups of 3 bits.
Each group of 3 bits records whether the following permissions are granted:
- read permission (most significant bit, on the left, decimal 4)
- write permission (second bit, in the middle, decimal 2)
- execute permission (least significant bit, on the right, decimal 1)
These are usually called "octal" rather than decimal, but I suspect you won't find any value in that extra complication, so you can just think of them as decimal numbers.
Permissions for files
The read and write permissions for files are pretty self-explanatory:
- If you have read permission for a file: the operating system kernel will allow you to read the contents of that file.
- If you have write permission for a file: the OS kernel will allow you to make changes to the contents of that file.
No permission implies any other permission. For example having write permission does not imply you have read permission, even though you might feel that you should.
The execute permission is more complicated.
Remember that on Linux file extensions don't make much difference. In Windows the operating system will attempt to execute anything with an .exe extension that you double-click on. Linux will refuse to execute any file which doesn't have execute permission.
On the surface it seems simple. You either are are are not allowed to execute a file. But the complications are in the details. What exactly does it mean to "execute" a file? Different people will have different answers to that question.
The easiest way to think about the execute permission on a file is as a hint: if this file has execute permissions: it is a program that's intended to be executed. If it doesn't have execute permissions: you are not meant to try to execute it, though if you really wanted to: you probably could figure out how to do it.
The read and write permissions are used to secure access to files. The execute permission is just a convenience.
Permissions for directories
Read, write, and execute permissions on directories are intuitive for some people, but for everyone they will make more sense if they begin their understanding by thinking of the concept of a file/directory on a filesystem.
Remember that there's a difference between the file's records (e.g. name, size, modification date, permissions) and the file's actual contents.
- For a file: its the contents are the bytes representing the text, image, or whatever data is in the file.
- For a directory: its contents are the records of the files/directories contained inside that directory. Not the contents of those files/directories, but only their records.
With that in mind:
- If you have the read permission on a directory: you will be allowed to read its contents, meaning: see what's inside the directory.
- If you have the write permission on a directory: you will be allowed to modify its contents, for example by copying a file into that directory, or deleting a file from that directory, or renaming a file in that directory.
As with files, the execute permission for directories is more complicated.
In most cases this is the simplest way to think of execute permissions:
- If you give a directory read or write permissions: give it execute permissions too.
- If you give a directory only execute permissions: you won't be able to read that directory's contents but can "get to" the directory's contents. For example:
- Open a file in that directory if you already know that file's name,
- Cd to a directory inside this directory, if you already know that directory's name
File ownership; user, group, others
On a POSIX filesystem each file record specifies a user who is the owner of that file, and a group who is the owner of that file.
The user who creates a file is automatically the owner of that file.
That user will be a member of a group, and by default the group owner of the created file will be that group.
Only the system administrator can change the owner of a file. The owner of the file can only change the group owner of a file if they are a member of the new group.
From an ownership/permissions point of view: if someone is not the owner, and they're not part of the group owner: they are classed as "others".
Putting it all together
Each file/directory record on your filesystem has 9 permission bits. The permission bits are shown when you run ls -l, but instead of showing 1s and 0s it shows the meaning of those bits:
- r for read, w for write, and x for execute if that permission is granted
- - if that permission is denied
Permissions for files
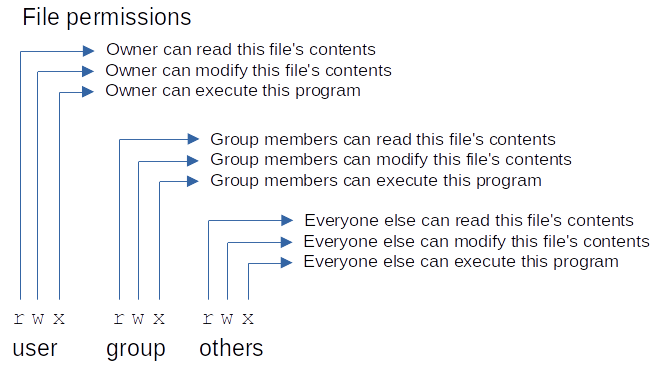
Permissions for directories
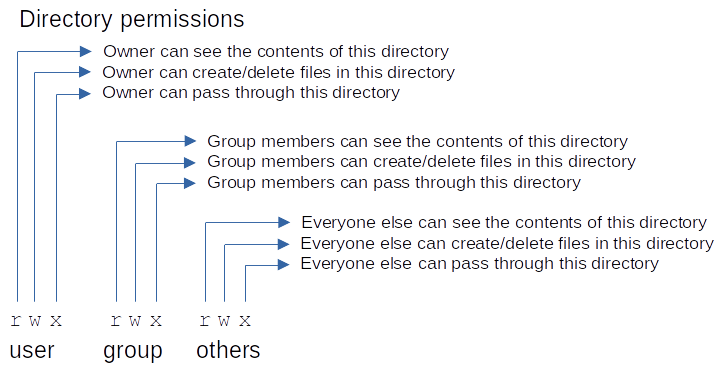
Examples
- Download this tarball for practice in this lab SamplePermissions.tar.xz and extract it into your downloads directory so that you'll end up with a ~/Downloads/SamplePermissions directory.
- Create another user on your workstation. The extra user will also help with the practice:
sudo adduser joe --uid 1145 # put in your own password at the sudo prompt # put in any password you like for the new user joe # press enter to accept the defaults for all the other questions
- In a terminal change to that directory, and run ls -l
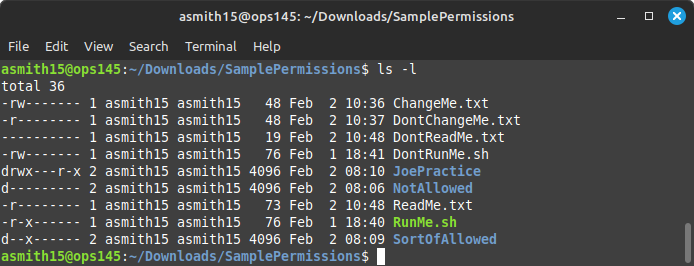
- Ignore for now the first character in the ls -l output, it has nothing to do with permissions.
- Here are examples of ways to check whether permissions set on these files work:
- Run cat nameofthefile to read a file's contents.
- Open the text file in a graphical text editor, make some changes, and attempt to save your changes.
- Run ./nameofthescript.sh to attempt to execute that script.
- Run mv originalnameoffile newnameoffile to attempt to rename a file or directory.
- Answer the following questions first, and then check whether you were correct.
- Which of the files can you read?
- Which of the files can you change the contents of?
- If you are unable to change the contents of a file, can you still rename that file? Does that change its permissions?
- Which of the files can you execute?
- Which subdirectories can you cd into?
- Which subdirectories can you see the contents of?
- Both the NotAllowed and SortOfAllowed directories contain a file called ForThoseWhoKnow.txt - can you read its contents?
- Open a second terminal window, change to the SamplePermissions directory, and switch to the joe user in that terminal using the su command:In that one window you are not your regular user, you are joe and you only have the privileges that joe has.
cd ~/Downloads/SamplePermissions su joe
- As joe, change to the JoePractice directory. Answer the following questions first, and then check whether you were correct.
- Who owns all the contents of this directory?
- Which of the files can you read? Which of the files can Joe read?
- Are there any files joe can read but you cannot?
- Close the second terminal.
You are the owner of the files, and joe (not being part of your group) has the permission of others.
Changing permissions with chmod using octal notation
Once you understand how permissions work: you can start changing them to accomplish security goals. The POSIX permissions system is relatively rudimentary, but it can still be used on its own to secure files on a server which is accessed by many people.
Permissions are changed with the chmod command. One way to use it is to specify new permissions using a 3-digit numeric notation.
Remember you have 9 permission bits, in 3 groups of 3. Each group of 3 bits is represented as a decimal number ranging from 0 to 7. When you need come up with a numeric permission to use with chmod: you have to do 3 sets of binary conversions and additions in your head.
Here's an example of how you would turn the permissions you want to set on a file into a numeric notation you can use with chmod:
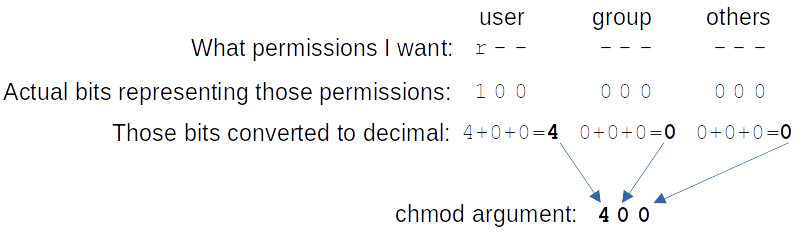
Here's another example with a different set of permissions, following exactly the same procedure:
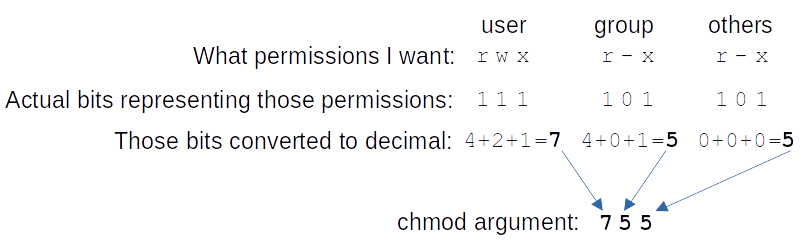
- Create a directory named lab4 inside your home directory.
- Use the graphical file manager and graphical archive manager to extract another copy of the SampleFiles directory and all its contents into ~/lab4
- Change your present working directory to ~/lab4/SamplePermissions in your terminal.
- Use chmod to allow yourself [you are the file owner] to read the file DontReadMe.txt:
chmod 400 DontReadMe.txt
- Test (using cat) that your new permissions work as expected.
- Use chmod to allow yourself to read and write to the file DontChangeMe.txt:
chmod 600 DontChangeMe.txt
- Test (using a graphical text editor) that your new permissions work as expected.
- Use chmod to allow yourself to read and write and execute the file DontRunMe.sh:
chmod 700 DontRunMe.sh
- Test that your new permissions work as expected by executing the DontRunme.sh script.
- Use chmod to allow yourself to see the contents of the SortOfAllowed directory:
chmod 500 SortOfAllowed/
- Test (using ls) that your new permissions work as expected.
- Use chmod to allow yourself to see and modify the contents of the SortOfAllowed directory:
chmod 700 NotAllowed/
- Test (using ls) that you can see the contents of NotAllowed, and test (using cp or mv or mkdir) that you can make changes to the contents of NotAllowed.
Note that in all the permissions above only the owner gets some particular permissions. The group and everyone else get no permissions (0). You can check that by trying to read or execute any of these files as the user joe.
- Keep your PWD as ~/lab4/SamplePermissions and use chmod to allow everyone who's not you (including joe) to read the file DraftToBoss.txt:
chmod 604 JoePractice/DraftToBoss.txt
- Open a second terminal, cd to ~/lab4/SamplePermissions/JoePractice and switch to the joe user in that second terminal.
- Confirm (using cat) that joe can now read the DraftToBoss.txt file.
Changing permissions with chmod using symbolic notation
Specifying permissions as a number is very efficient if you know exactly what nine permissions you want the file/directory to have.
Sometimes you just need to change one particular permission, in which case it's easier to use the symbolic notation. The chmod command is invoked the same way, but instead of the numeric permissions it's a combination of letter codes:
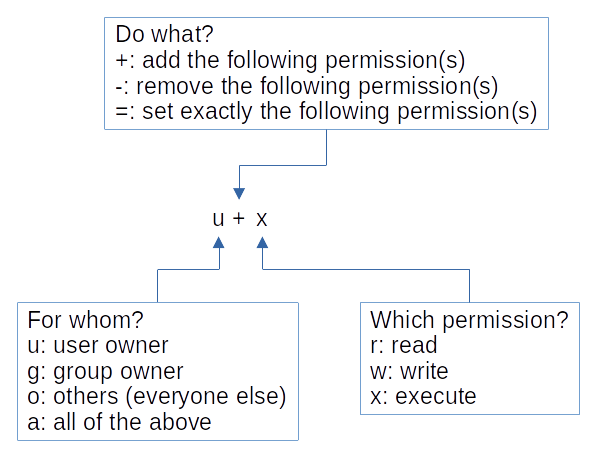
There are too many combinations to remember at this time, so we'll just look at a couple of examples.
- In your terminal change to ~/lab4/SamplePermissions and run ls -l to confirm that the group has no permissions to any files or directories in there:
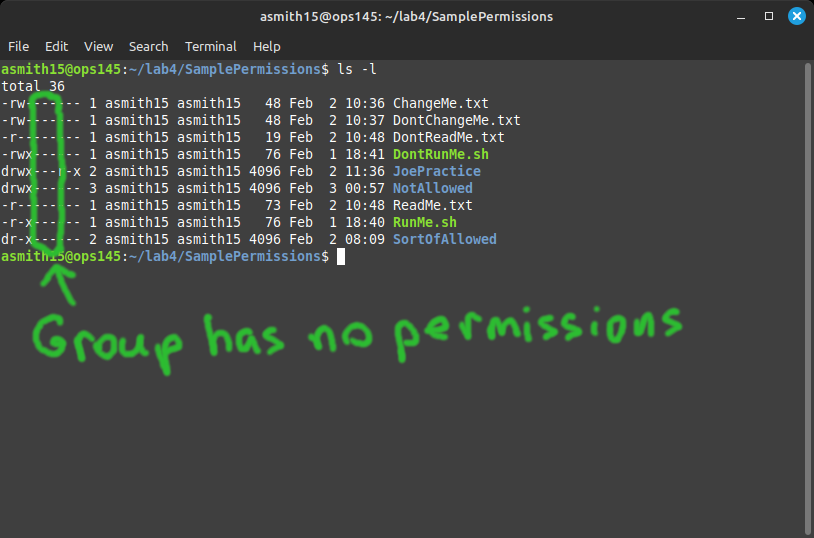
- Use the chmod command with symbolic permissions to give the group owner execute permissions for the shell scripts, without modifying any of the other permissions:Note that you can change permissions on more than one file at the same time, as long as you want the same thing done to all the files.
chmod g+x RunMe.sh DontRunMe.sh
- Confirm using ls -l that the permissions were changed as you exptected.
- Use the chmod command with symbolic permissions to give the group owner read permissions for all the text files, without modifying any of the other permissions:The *.txt is a little new: it's the same * wildcard you've used in the last lab. We'll spend more time on wildcards in a later lab.
chmod g+r *.txt
- Confirm using ls -l that the permissions were changed as you exptected.
- Use the chmod command with symbolic permissions to give the group owner read and write and execute permissions to the JoePractice and NotAllowed directories, without modifying any of the other permissions:
chmod g+rwx JoePractice/ NotAllowed/
- Confirm using ls -l that the permissions were changed as you exptected.
Your permissions should look like this by now:
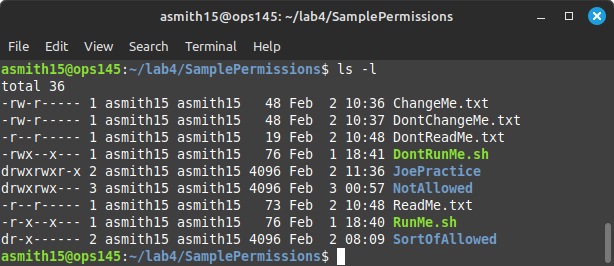
In many environments the group owner typically has the same permissions for files as the user owner. But that sort of decision needs to be made on a case-by-case basis.
Submit evidence of your work
After you finish the lab: run the following commands to submit your work:
cd ~
wget http://ops345.ca/check/ops145-lab4-check.sh
chmod 700 ops145-lab4-check.sh
./ops145-lab4-check.sh
If it says "Your lab4 has been submitted": make a screenshot, and you're done. If it gives you any warnings or errors: you have to fix them and try the ./ops145-lab4-check.sh command again.